
Unlock Your Selenium WebDriver Knowledge: Interview Questions and Answers Explained
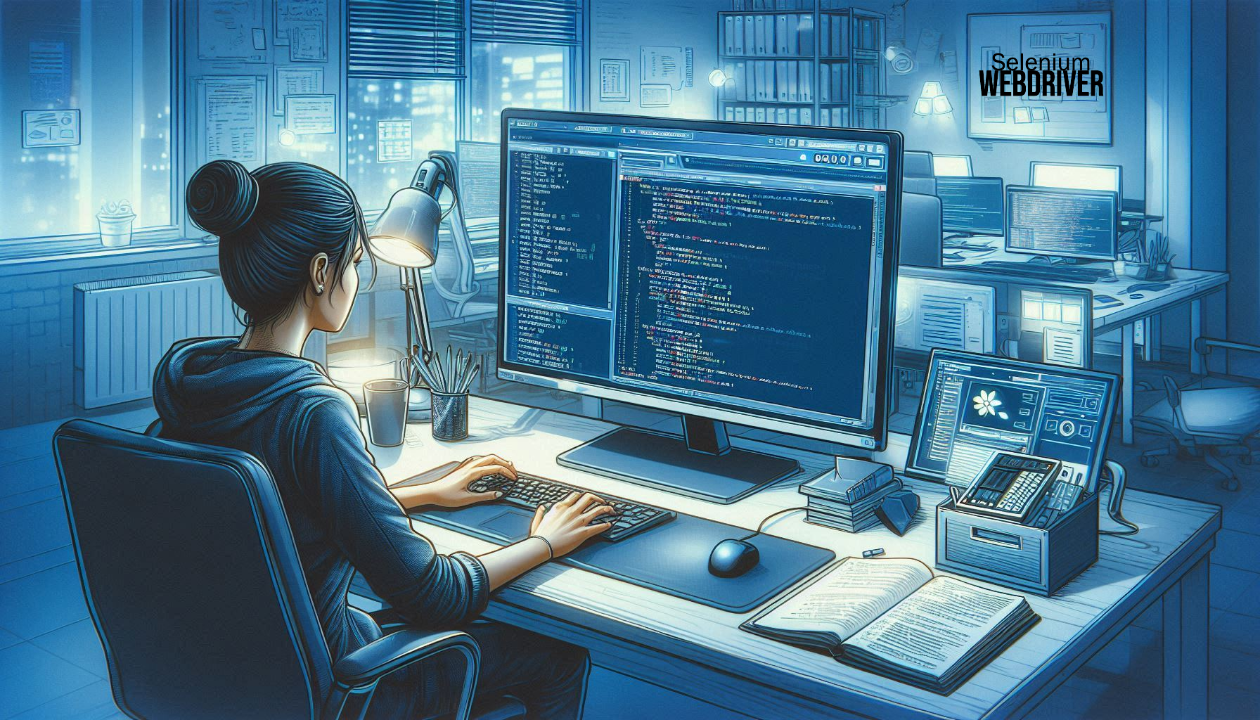
1. What is Selenium WebDriver?
Answer: Selenium WebDriver is an open-source automation testing tool used for automating web applications across various browsers. Unlike Selenium RC (Remote Control), WebDriver directly interacts with the browser without the need for a server. WebDriver supports various programming languages like Java, C#, Python, Ruby, and JavaScript, and allows interaction with browser elements like buttons, text fields, links, etc.
2. What are the different components of Selenium?
Answer: The Selenium suite consists of several components:
- Selenium WebDriver: The core part used for browser automation.
- Selenium IDE: A Firefox plugin used for creating scripts without programming.
- Selenium Grid: Allows for distributed testing across multiple machines and browsers.
- Selenium RC (Remote Control): An older component, now largely replaced by WebDriver.
3. How does Selenium WebDriver interact with browsers?
Answer: WebDriver interacts with browsers using browser-specific drivers. Each browser has its own WebDriver implementation (e.g., ChromeDriver for Google Chrome, GeckoDriver for Firefox). WebDriver sends commands to the browser using the browser driver, which then executes the commands in the browser and returns the results.
4. What is the difference between WebDriver and Selenium RC?
Answer:
- WebDriver directly communicates with the browser and does not require a server for execution.
- Selenium RC requires a server to send commands and retrieve results from the browser. WebDriver is faster and more reliable as it interacts directly with the browser’s native support.
5. What are some key advantages of Selenium WebDriver?
Answer:
- Cross-browser support: Works with multiple browsers like Chrome, Firefox, Safari, Edge, etc.
- Multi-language support: Supports Java, C#, Python, Ruby, JavaScript, and Kotlin.
- Faster execution: Executes faster compared to Selenium RC because it directly interacts with the browser.
- Rich set of APIs: Provides a variety of methods to perform actions on web elements.
- No need for a server: Unlike Selenium RC, WebDriver doesn't require a separate server to communicate with the browser.
6. What is the purpose of the findElement() method in Selenium WebDriver?
Answer: The findElement() method is used to locate a web element on a webpage. It accepts a locator strategy (e.g., ID, name, class, XPath, etc.) and returns the first element that matches the locator. If no matching element is found, it throws a NoSuchElementException.
7. What is a locator in Selenium WebDriver?
Answer: A locator is a strategy used to find an element on a webpage. Some common locator strategies include:
- ID: driver.findElement(By.id("elementId"))
- Name: driver.findElement(By.name("elementName"))
- Class Name: driver.findElement(By.className("className"))
- Tag Name: driver.findElement(By.tagName("tagName"))
- CSS Selector: driver.findElement(By.cssSelector("cssSelector"))
- XPath: driver.findElement(By.xpath("xpathExpression"))
8. What is the WebElement interface in Selenium WebDriver?
Answer: WebElement is an interface in Selenium WebDriver that represents an HTML element. It provides methods to interact with the elements, such as clicking, sending keys, getting text, and retrieving attributes. Examples of methods are:
- click()
- sendKeys()
- getText()
- getAttribute()
9. How do you handle pop-ups in Selenium WebDriver?
Answer: Selenium WebDriver can handle pop-ups using the Alert interface. The Alert interface provides methods to accept, dismiss, retrieve text, and interact with alerts.
- To switch to the alert: Alert alert = driver.switchTo().alert();
- To accept the alert: alert.accept();
- To dismiss the alert: alert.dismiss();
- To get the text of the alert: String alertText = alert.getText();
10. What is implicit wait in Selenium WebDriver?
Answer: Implicit wait is used to tell the WebDriver to wait for a specified amount of time before throwing a NoSuchElementException if an element is not found immediately. It is applied globally across all elements.
java
CopyEdit
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
11. What is explicit wait in Selenium WebDriver?
Answer: Explicit wait is used for waiting for a specific condition to be true before proceeding with the execution of the script. It is more specific and can be applied to individual elements.
java
CopyEdit
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
12. How do you handle drop-downs in Selenium WebDriver?
Answer: Selenium WebDriver provides the Select class to interact with dropdowns. You can select options by visible text, index, or value.
- Select by visible text:
java
CopyEdit
Select select = new Select(driver.findElement(By.id("dropdown")));
select.selectByVisibleText("Option Text");
- Select by index:
java
CopyEdit
select.selectByIndex(2);
13. What is the difference between selectByVisibleText() and selectByValue()?
Answer:
- selectByVisibleText(): Selects an option from a drop-down based on the visible text of the option.
- selectByValue(): Selects an option from a drop-down based on the value attribute of the option.
14. How can you handle dynamic web elements in Selenium WebDriver?
Answer: To handle dynamic web elements, you can use explicit waits with dynamic XPath or CSS selectors. The key is to wait for the element to be present, visible, or clickable before interacting with it.
15. What is the purpose of driver.get() in Selenium?
Answer: The driver.get() method is used to launch a web page in the specified browser. It takes a URL as an argument and navigates to that URL.
java
CopyEdit
driver.get("https://www.example.com");
16. What is the driver.quit() method in Selenium?
Answer: driver.quit() is used to close the browser and end the session. It terminates the WebDriver instance, shutting down the browser completely.
17. How do you handle mouse events in Selenium WebDriver?
Answer: Selenium WebDriver provides the Actions class for handling mouse events like mouse hover, right-click, and drag-and-drop.
- Example of mouse hover:
java
CopyEdit
Actions action = new Actions(driver);
WebElement element = driver.findElement(By.id("menu"));
action.moveToElement(element).perform();
18. How can you perform a right-click operation in Selenium WebDriver?
Answer: You can perform a right-click operation using the Actions class as follows:
java
CopyEdit
Actions action = new Actions(driver);
WebElement element = driver.findElement(By.id("rightClickElement"));
action.contextClick(element).perform();
19. What is the driver.navigate() method used for?
Answer: The driver.navigate() method is used for navigating between pages or moving forward and backward in the browser history. It is often used for navigating URLs or moving between pages in the same session.
- To go back:
java
CopyEdit
driver.navigate().back();
- To go forward:
java
CopyEdit
driver.navigate().forward();
20. What is the purpose of driver.getCurrentUrl() in Selenium?
Answer: The getCurrentUrl() method is used to get the URL of the current page. It returns the URL as a string.
java
CopyEdit
String currentUrl = driver.getCurrentUrl();
21. How do you take a screenshot in Selenium WebDriver?
Answer: Selenium provides the TakesScreenshot interface for capturing screenshots.
java
CopyEdit
TakesScreenshot ts = (TakesScreenshot) driver;
File source = ts.getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(source, new File("screenshot.png"));
22. What is the driver.switchTo() method in Selenium WebDriver?
Answer: driver.switchTo() is used to switch between different frames, windows, or alerts. It allows interaction with elements inside iframes or different browser windows.
- To switch to a frame:
java
CopyEdit
driver.switchTo().frame("frameName");
- To switch to an alert:
java
CopyEdit
driver.switchTo().alert();
23. What is a WebDriver Wait?
Answer: A WebDriver wait is a mechanism that allows you to pause execution until a certain condition is met (e.g., an element becoming visible). It's used to handle timing issues when dealing with dynamic web pages.
24. How do you find multiple elements in Selenium WebDriver?
Answer: You can use the findElements() method to locate multiple elements matching a locator. It returns a list of WebElement objects.
java
CopyEdit
List<WebElement> elements = driver.findElements(By.className("elementClass"));
25. What are the advantages of using Selenium WebDriver over Selenium IDE?
Answer:
- Selenium WebDriver allows writing code in multiple programming languages, whereas Selenium IDE is limited to the use of its own scripting language.
- WebDriver supports complex test scripts, integration with testing frameworks, and continuous integration, which are not possible with Selenium IDE.
26. What is the role of driver.manage().timeouts().implicitlyWait()?
Answer: This method sets the default waiting time for all elements in the WebDriver. It instructs the WebDriver to wait for a certain amount of time before throwing an exception when an element is not found.
27. What is the JavaScriptExecutor interface used for in Selenium WebDriver?
Answer: The JavaScriptExecutor interface allows you to execute JavaScript code within the context of the browser. This is useful when you need to interact with elements that cannot be interacted with directly through WebDriver.
java
CopyEdit
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0,250)");
28. How can you handle SSL certificate warnings in Selenium WebDriver?
Answer: SSL certificate errors can be handled by configuring the browser’s profile to ignore SSL warnings.
- Example for Chrome:
java
CopyEdit
ChromeOptions options = new ChromeOptions();
options.addArguments("--ignore-certificate-errors");
WebDriver driver = new ChromeDriver(options);
29. What is Page Object Model (POM) in Selenium?
Answer: Page Object Model is a design pattern in Selenium where you create a separate class for each page of the web application. Each class represents a page, and it contains methods to interact with the elements on that page. This promotes code reuse and makes the tests more maintainable.
30. How can you handle frames in Selenium WebDriver?
Answer: To switch to a frame in Selenium, you use the switchTo().frame() method.
java
CopyEdit
driver.switchTo().frame("frameName");
// Perform operations inside the frame
driver.switchTo().defaultContent(); // Switch back to the main content