
Understanding Java Object-Oriented Programming (OOP) Concepts
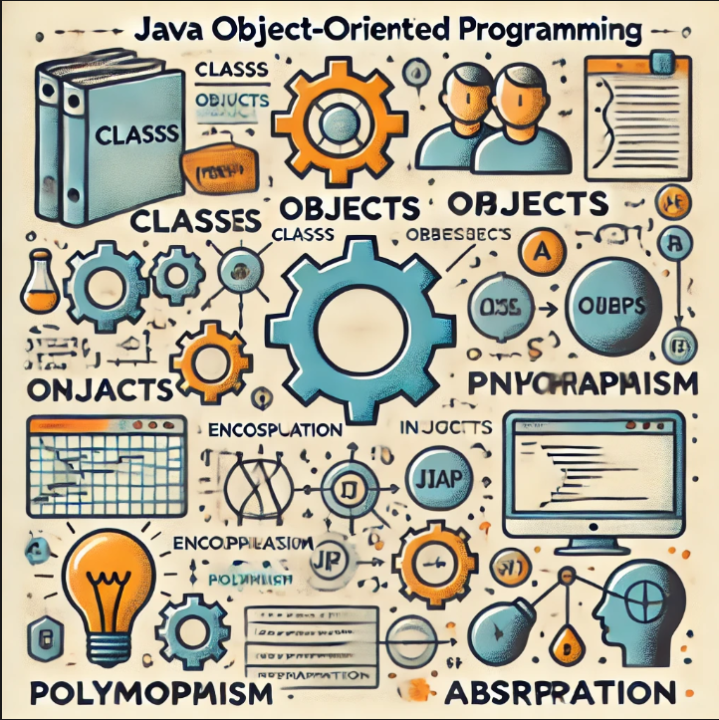
Java is a powerful and widely used programming language known for its versatility and robustness. One of the core features that sets Java apart from many other programming languages is its Object-Oriented Programming (OOP) paradigm. OOP is a programming style that organizes software design around data, or objects, rather than functions and logic. This article explores the key OOP concepts in Java, providing insights into how they enhance software development.
1. Classes and Objects
Classes are blueprints for creating objects. They define the properties (attributes) and behaviors (methods) that the objects created from the class can have. In Java, a class is defined using the class keyword.
Objects are instances of classes. When you create an object from a class, you are creating an entity that possesses the characteristics defined by the class.
Example:
class Car { String color; String model; void displayDetails() { System.out.println("Car model: " + model + ", Color: " + color); } } public class Main { public static void main(String[] args) { Car myCar = new Car(); myCar.color = "Red"; myCar.model = "Toyota"; myCar.displayDetails(); // Output: Car model: Toyota, Color: Red } }
2. Encapsulation
Encapsulation is the concept of bundling the data (attributes) and methods that operate on the data into a single unit, known as a class. This helps in restricting direct access to some components of an object and is achieved through access modifiers.
Java provides four access modifiers:
- public: Accessible from any other class.
- private: Accessible only within the class it is declared.
- protected: Accessible within the same package and subclasses.
- default: Accessible only within the same package.
By using encapsulation, you can control how the data in your objects is accessed and modified.
Example:
class Account { private double balance; // private attribute public void deposit(double amount) { balance += amount; } public double getBalance() { return balance; } } public class Main { public static void main(String[] args) { Account myAccount = new Account(); myAccount.deposit(1000); System.out.println("Balance: " + myAccount.getBalance()); // Output: Balance: 1000.0 } }
3. Inheritance
Inheritance is a mechanism that allows one class to inherit properties and methods from another class. This promotes code reusability and establishes a relationship between classes. In Java, a class can extend another class using the extends keyword.
Example:
class Vehicle { void start() { System.out.println("Vehicle is starting"); } } class Bike extends Vehicle { void ringBell() { System.out.println("Bike bell rings"); } } public class Main { public static void main(String[] args) { Bike myBike = new Bike(); myBike.start(); // Output: Vehicle is starting myBike.ringBell(); // Output: Bike bell rings } }
4. Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. It is primarily of two types: compile-time polymorphism (method overloading) and runtime polymorphism (method overriding).
- Method Overloading: Defining multiple methods with the same name but different parameters in the same class.
- Method Overriding: Redefining a method in a subclass that already exists in the parent class.
Example of Method Overloading:
class MathOperations { int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; } } public class Main { public static void main(String[] args) { MathOperations math = new MathOperations(); System.out.println(math.add(5, 10)); // Output: 15 System.out.println(math.add(5.5, 10.5)); // Output: 16.0 } } Example of Method Overriding: class Animal { void sound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { void sound() { System.out.println("Dog barks"); } } public class Main { public static void main(String[] args) { Animal myDog = new Dog(); myDog.sound(); // Output: Dog barks } }
5. Abstraction
Abstraction is the concept of hiding complex implementation details and showing only the essential features of an object. In Java, abstraction can be achieved using abstract classes and interfaces.
- Abstract Classes: A class that cannot be instantiated and may contain abstract methods (without implementation) and concrete methods (with implementation).
- Interfaces: A contract that defines a set of methods that implementing classes must provide.
Example of an Abstract Class:
abstract class Shape { abstract void draw(); } class Circle extends Shape { void draw() { System.out.println("Drawing a circle"); } } public class Main { public static void main(String[] args) { Shape myShape = new Circle(); myShape.draw(); // Output: Drawing a circle } }
Example of an Interface:
interface Animal { void eat(); } class Cat implements Animal { public void eat() { System.out.println("Cat eats"); } } public class Main { public static void main(String[] args) { Animal myCat = new Cat(); myCat.eat(); // Output: Cat eats } }
Conclusion
Java's Object-Oriented Programming concepts—classes and objects, encapsulation, inheritance, polymorphism, and abstraction—provide a powerful framework for developing modular and reusable code. Understanding these concepts is essential for any Java developer and forms the backbone of effective software design. By leveraging OOP principles, developers can create applications that are easier to maintain, extend, and understand, ultimately leading to more robust software solutions.