
Test Driven Development (TDD): A Complete Guide for Software Quality and Agile Success
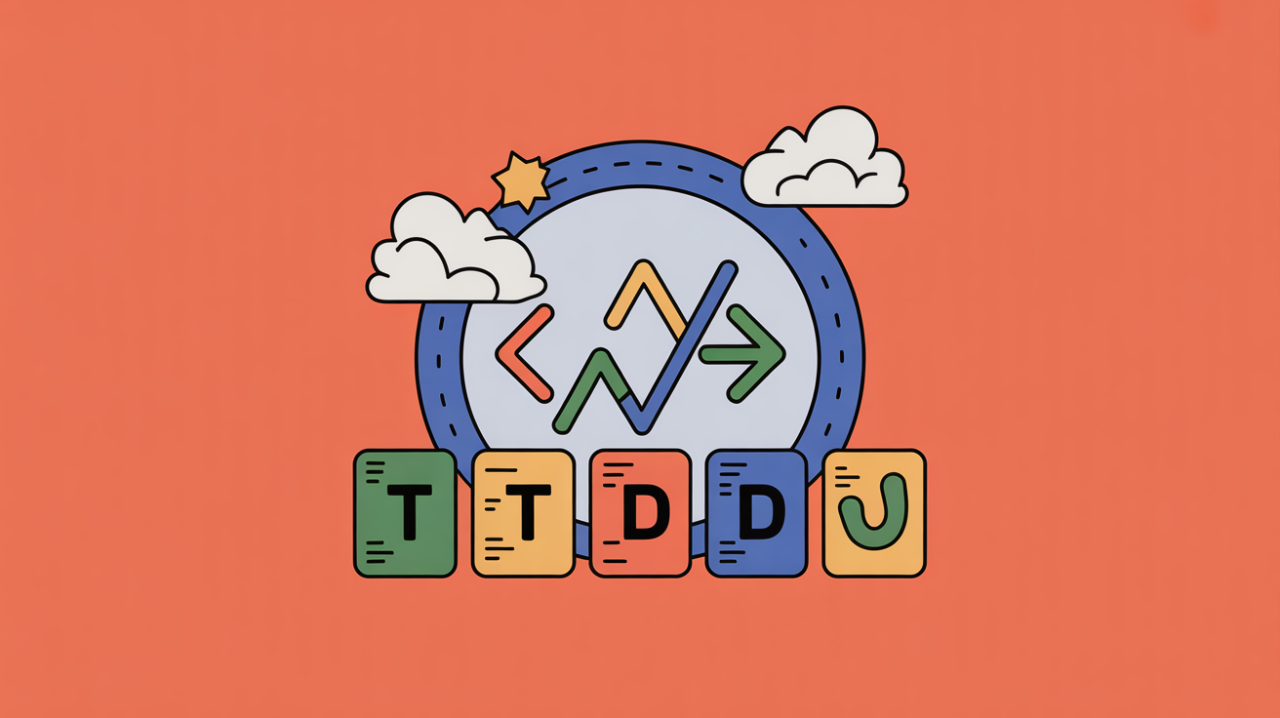
If there’s one development approach that’s fundamentally changed how I write and think about code, it’s Test Driven Development (TDD). As a senior QA engineer who’s worked across various Agile teams and tech stacks, I can say from firsthand experience that TDD is more than just a testing strategy—it’s a mindset that shapes the architecture and maintainability of the software we build.
I’ll walk you through what TDD is, how it works, and why it’s become an essential part of my software development toolbox. I’ll also share practical tips, tools, benefits, and common pitfalls—everything you need to either get started or go deeper into your TDD journey.
What is Test Driven Development (TDD)?
At its core, Test Driven Development (TDD) is a software development practice where you write tests before you write the code that makes those tests pass. It flips the traditional approach on its head. Instead of writing code and testing it afterward, you define the expected behavior first via a failing test—then write the code to make it pass.
The process follows a tight loop known as Red-Green-Refactor:
- Red – Write a test that fails.
- Green – Write just enough code to pass the test.
- Refactor – Clean up the code while keeping the test passing.
This cycle is repeated until the feature or unit is complete. I first encountered TDD while working on an Agile team practicing Extreme Programming (XP), and although it was a bit uncomfortable at first, it completely transformed my coding discipline.
The Core Principles of TDD
TDD is simple to understand but challenging to master. The key lies in sticking to the Red-Green-Refactor loop. Here's how I approach it on a day-to-day basis:
- I never write a line of production code unless I have a failing test to justify it.
- I keep my tests small, focused on a single responsibility.
- Refactoring isn't just about cleaning code—it's about finding better abstractions without changing the behavior.
This discipline forces me to think about the design before implementation, which leads to fewer bugs and better software structure. It took practice, but it became second nature over time.
The TDD Process: Step-by-Step
Let me walk you through the typical TDD cycle using an example from my experience building a simple feature in a REST API:
Step 1: Understand the Requirement
Before touching the code, I clarify what the feature needs to do. For instance, if I’m building a function to calculate a user’s age based on their date of birth, I define the expected behavior clearly.
Step 2: Write the First Test (Red)
I write a unit test that calls the function with a specific date and expects a result. Since the function doesn’t exist yet, the test fails.
Step 3: Run the Test and Watch it Fail
That failure is confirmation that the test is valid and that the behavior hasn't been implemented yet.
Step 4: Write Minimal Code to Pass (Green)
I then write just enough code to pass the test—nothing more. Maybe that means returning a hardcoded value at first.
Step 5: Refactor (While Keeping Green)
Once the test passes, I improve the code—perhaps replacing the hardcoded value with a real calculation.
Step 6: Repeat
I add more tests for edge cases, continue refining the function, and evolve the design with confidence because tests guard my back.
This process is fast, iterative, and safe. It forces me to keep my code modular and testable.
Benefits of Test Driven Development
TDD isn’t just about testing—it’s about quality from the ground up. Here are the biggest benefits I’ve experienced:
1. Improved Code Quality
Since I’m always thinking in terms of test cases, the resulting code is inherently more reliable. I’ve seen a drastic drop in production bugs since adopting TDD.
2. Cleaner Architecture
TDD nudges you toward writing smaller, decoupled components. This has led to better abstractions and lower technical debt in every project I’ve worked on.
3. Confidence in Refactoring
With a suite of tests in place, I can safely refactor without the fear of breaking functionality. That confidence has been invaluable, especially in legacy codebases.
4. Better Documentation
Tests act as living documentation. New developers on my teams have often found it easier to understand code by reading the tests than reading lengthy docs.
5. Improved Developer Focus
TDD helps me focus on one small piece of functionality at a time. It keeps me grounded in solving the problem, not getting distracted by implementation details.
Challenges and Limitations of TDD
TDD is powerful, but it’s not perfect. Here are some of the challenges I’ve faced:
- Learning Curve: When I first started, writing tests before code felt backward. It took real effort to build the habit.
- Initial Time Investment: Writing tests upfront slows things down in the short term, but in my experience, it pays off tenfold in the long run.
- False Sense of Coverage: TDD covers units well but doesn’t replace the need for integration or UI testing.
- Not Always Practical: In UI-heavy apps or exploratory development, TDD can feel restrictive or inefficient.
I’ve learned to assess whether TDD is the right tool for the job instead of blindly applying it everywhere.
TDD vs Traditional Development
In traditional development, I used to write a big chunk of code first, then write tests—if I remembered. That led to fragile, bug-prone systems.
With TDD, the approach is reversed and much more deliberate. Here's how they compare:
Best Practices for Effective TDD
Here are some practices I follow that have helped me get the most from TDD:
- Start small: Don’t try to test every detail at once. Focus on one behavior.
- Isolate tests: Avoid dependencies like databases or external APIs unless necessary.
- Name tests clearly: So the purpose is obvious at a glance.
- Run tests frequently: I run them after every small change.
- Refactor aggressively: Clean code is easier to test and extend.
- Collaborate: TDD works best when teams share ownership of test quality.
TDD Tools and Frameworks
Over the years, I’ve used different tools for TDD depending on the language:
- Java: JUnit, TestNG
- JavaScript/TypeScript: Jest is my go-to, especially with React apps
- Python: Pytest is elegant and powerful
- C#: NUnit and xUnit
- Ruby: RSpec makes testing almost enjoyable
Most modern IDEs integrate well with these tools and allow for real-time test feedback. Combine that with a CI tool like Jenkins or GitHub Actions, and you’ve got a seamless TDD workflow.
Real-World Example of TDD in Action
One of the first real projects where I applied TDD from scratch was building a user authentication module. I started by writing tests like:
- Should return success when the username and password are correct
- Should return error when the password is wrong
- Should lock the account after three failed attempts
I didn’t touch the implementation until these tests were written. As I built out the logic, each new test guided my next step. The result was a clean, secure authentication module with almost zero post-deployment bugs.
The most satisfying part? Debugging took minutes instead of hours because every function was well-tested and predictable.
TDD in Agile and DevOps Culture
TDD fits perfectly into Agile and DevOps. In my Agile teams, we’ve used TDD as part of every sprint. It complements practices like:
- CI/CD: Tests act as gatekeepers before code hits production
- Pair Programming: Writing tests together reveals edge cases early
- Behavior Driven Development (BDD): TDD is technical, BDD focuses on business behavior. They work beautifully together.
TDD also supports the "shift-left" approach in QA—catching bugs earlier in the pipeline where they’re cheaper to fix.
When NOT to Use TDD
I’m a fan of TDD, but I don’t apply it blindly. I avoid it in cases like:
- Rapid Prototyping: When speed and iteration matter more than safety
- Unstable Requirements: When business logic is changing too fast to keep tests up-to-date
- UI/UX Testing: Frontend animation and interaction logic is better handled with end-to-end or visual regression tests
TDD is a tool—not a religion.
Common Misconceptions About TDD
I’ve heard many myths about TDD, so let’s clear a few up:
- “TDD is just unit testing.”
- No—TDD is about designing your code through testing, not just writing tests.
- “TDD slows you down.”
- Maybe at first, but in the long run it speeds up debugging and maintenance.
- “You have to write all tests first.”
- Not true. You write tests iteratively—one at a time, just ahead of implementation.
- “TDD eliminates the need for other tests.”
- Definitely not. TDD is just one part of a full testing strategy.
Conclusion
Test Driven Development has fundamentally reshaped how I build and maintain software. It’s made me a more thoughtful developer and a more confident QA engineer. While it’s not a silver bullet, it’s a powerful tool when used wisely.
If you’re new to TDD, start small. Pick a module or component, and give it a shot. If you're experienced, challenge yourself to write even better tests that truly drive design.
The real beauty of TDD is in how it aligns quality, agility, and clarity—something every modern software team can benefit from.
FAQ: Test Driven Development (TDD)
What is Test Driven Development (TDD)?
TDD is a software development technique where tests are written before the code itself, following a cycle of Red-Green-Refactor.
What are the benefits of TDD?
TDD improves code quality, design, test coverage, and developer confidence while reducing bugs and enabling safer refactoring.
Is TDD the same as unit testing?
No. While TDD involves unit tests, it is a development methodology—not just a testing practice.
Which languages support TDD?
TDD can be practiced in most languages including Java, Python, JavaScript, C#, and Ruby.
Can I use TDD in Agile development?
Yes, TDD fits perfectly into Agile and DevOps workflows and enhances continuous integration and quality assurance practices.
When should I avoid TDD?
Avoid TDD in cases like prototyping, volatile requirements, or heavily UI-based systems where unit tests offer limited value.