
Radio Buttons in Selenium: A Comprehensive Guide to Web Testing 🔘
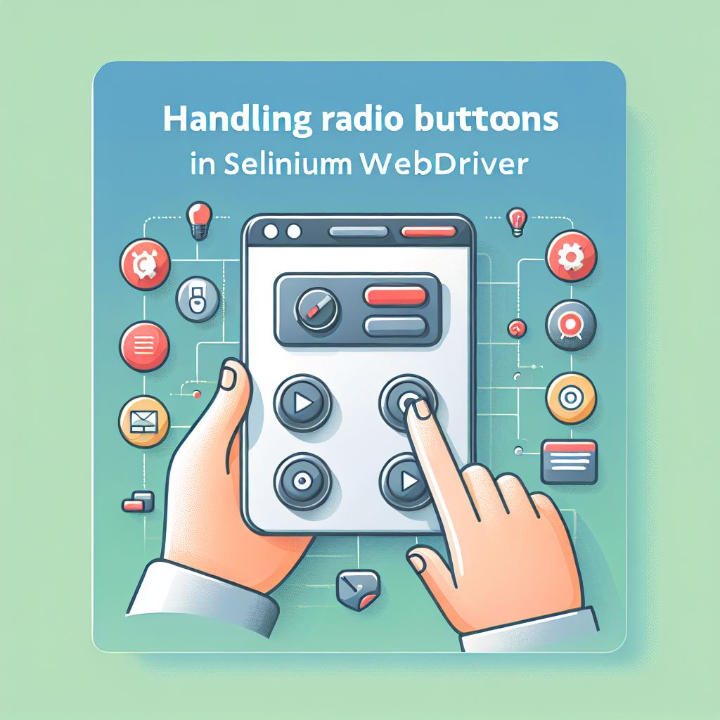
Introduction
In web testing 🌐, UI elements such as buttons, checkboxes✅ , and radio buttons 🔘 play a critical role in user interaction. For testers, ensuring that these elements function correctly is essential. This blog will focus on automating the testing of radio buttons using Selenium, one of the most popular frameworks for web automation testing🤖.
What are Radio Buttons?
Radio buttons are interactive elements commonly used in web forms that allow users to select one option from a predefined list📋. Unlike checkboxes, which allow for multiple selections, a radio button allows only one option to be selected at a time. Understanding how to test these elements efficiently is key to ensuring a smooth user experience🌟.
How to Identify Radio Buttons in Selenium 🔍
Before automating the testing of radio buttons, you must identify them on the web page. Selenium provides various locators such as ID, Name, Class Name, XPath, and CSS Selector to target radio buttons. For example:
// Locate a radio button by its name attribute
WebElement radioButton = driver.findElement(By.name("gender"));
In this example, the findElement method locates the radio button by its name attribute. You can replace "gender" with the appropriate name or any other locator strategy that works best for your web page🔧.
Automating Radio Button Selection 🖱️
Once identified, you can automate the process of selecting a radio button using the click() method in Selenium. Here's an example:
// Locate and select the radio button
WebElement radioButton = driver.findElement(By.id("male"));
radioButton.click();
In this case, the radio button with the id attribute "male" will be selected when the test runs🎯.
Verifying the Selection ✅
It is important to verify whether the correct radio button has been selected during testing. Selenium allows you to check if a radio button is selected using the isSelected() method:
// Verify if the radio button is selected
boolean isSelected = radioButton.isSelected();
System.out.println("Radio Button Selected: " + isSelected);
If the method returns true, the radio button is selected; otherwise, it's not✅.
Testing Multiple Radio Buttons 🔄
In scenarios where multiple radio buttons are present (for example, "Male" and "Female"), it’s essential to ensure that only one radio button can be selected at a time. Selenium can help automate this process:
// Select the first radio button
WebElement maleRadioButton = driver.findElement(By.id("male"));
maleRadioButton.click();
// Verify that only one radio button is selected
WebElement femaleRadioButton = driver.findElement(By.id("female"));
assert !femaleRadioButton.isSelected() : "Female radio button should not be selected";
By doing this, you ensure that only one choice is selected, even if there are multiple radio buttons 🔒.
Handling Disabled Radio Buttons 🚫
Sometimes, radio buttons can be disabled under certain conditions. In such cases, Selenium’s isEnabled() method can be used to verify that the radio button is disabled:
// Verify if the radio button is enabled
WebElement radioButton = driver.findElement(By.id("other"));
boolean isEnabled = radioButton.isEnabled();
System.out.println("Radio Button Enabled: " + isEnabled);
This ensures that you are testing for proper functionality, even when certain options are unavailable 🚷.
Conclusion 🎉
Testing radio buttons in web applications using Selenium is a fundamental skill for web testers 🧑💻. Through this blog, we've covered how to locate, select, verify, and handle radio buttons during automated web testing. By using Selenium's powerful methods, you can ensure that the user interface functions as expected, providing a seamless experience for the end-user 🚀.