
Mastering the Page Object Model (POM): Your Ultimate Guide to Test Automation
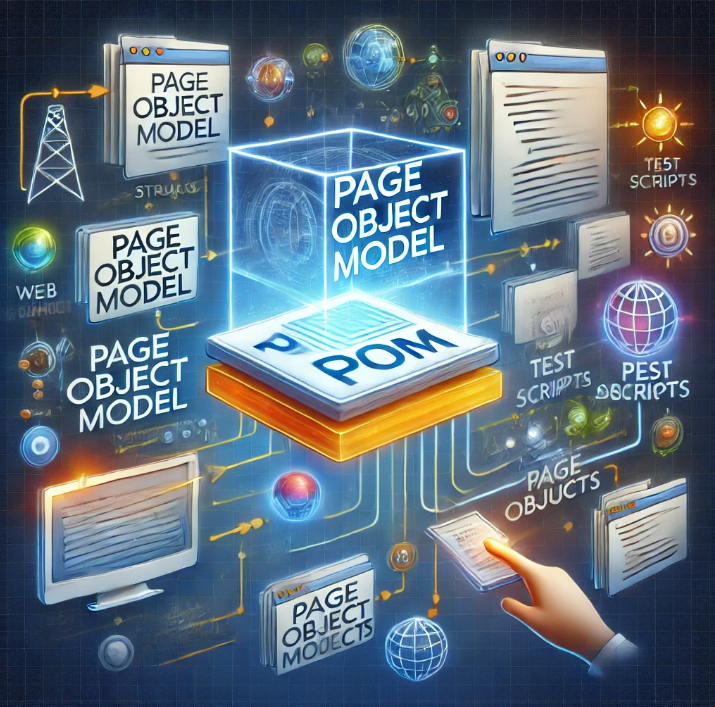
The Page Object Model (POM) is a design pattern widely used in test automation to enhance the readability, maintainability, and reusability of test scripts. It works by creating a layer of abstraction between the test scripts and the web application's user interface. In this pattern, each page of the application is represented as a separate class in the code, encapsulating its elements and interactions as methods.
What is Page Object Model (POM)?
POM is a framework that organizes test code by dividing it into:
- Page Classes: Representing web pages or components.
- Test Classes: Housing test cases that interact with the methods of the page classes.
For example, a login page may have its own class, which contains methods to input a username, input a password, and click the login button.
Key Features of POM
- Encapsulation of Web Elements:
- Each page's UI elements are defined within a page class, preventing duplication and making the test cases more intuitive.
- Reusability:
- Since each page has its methods and elements encapsulated, the code can be reused across multiple test cases.
- Abstraction:
- Test scripts don’t directly interact with the web elements. Instead, they use the methods provided in the page class, keeping test logic separate from UI logic.
- Scalability:
- POM makes it easier to scale test scripts as the application grows. New functionality can be accommodated by simply adding new page classes.
How Does POM Help?
1. Improved Code Maintainability
- If the UI changes, you only need to update the page class for the affected page, instead of updating all test scripts.
- For instance, if the ID of a login button changes, it needs to be updated only in the login page class.
2. Enhanced Test Readability
- POM makes test scripts easy to read by using descriptive method names in page classes.
A test for logging in might read:
LoginPage.enterUsername("user@example.com");
LoginPage.enterPassword("password123");
LoginPage.clickLoginButton();
3. Separation of Concerns
The separation between test logic and UI interactions ensures that tests remain focused on business logic, not implementation details.
4. Reusability Across Tests
- A single page class can be reused in multiple test cases, reducing code duplication and improving consistency.
5. Ease of Debugging
- If a test fails, the issue can often be pinpointed to a specific page or method, speeding up debugging.
Example of POM in Action
Scenario: Testing the login functionality of a web application.
LoginPage Class:
public class LoginPage {
WebDriver driver;
// Web elements
By usernameField = By.id("username");
By passwordField = By.id("password");
By loginButton = By.id("login");
// Constructor
public LoginPage(WebDriver driver) {
this.driver = driver;
}
// Page actions
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordField).sendKeys(password);
}
public void clickLoginButton() {
driver.findElement(loginButton).click();
}
}
Test Script:
public class LoginTest { WebDriver driver = new ChromeDriver(); LoginPage loginPage = new LoginPage(driver); @Test public void testSuccessfulLogin() { driver.get("https://example.com/login"); loginPage.enterUsername("user@example.com"); loginPage.enterPassword("password123"); loginPage.clickLoginButton(); // Assert successful login Assert.assertEquals(driver.getTitle(), "Dashboard"); } }
Real-Life Benefits of Using POM
- Dynamic Web Applications:
- As web applications grow and evolve, POM ensures that maintaining automation scripts is manageable.
- Team Collaboration:
- Multiple testers and developers can work on different page classes simultaneously, streamlining team efforts.
- Consistency Across Tests:
- Standardizing how pages are interacted with makes the test suite consistent and professional.
- Adaptability for Continuous Testing:
- POM is well-suited for Agile and DevOps practices, where testing must be continuous and adaptable to frequent changes.
Conclusion
The Page Object Model is a cornerstone of efficient test automation. By promoting organization, abstraction, and reusability, POM helps QA teams build scalable and maintainable test suites. For modern software development, where applications undergo frequent updates, adopting POM can significantly reduce the testing effort while ensuring high-quality releases.