
Contract Testing with Pact & API Automation Strategies
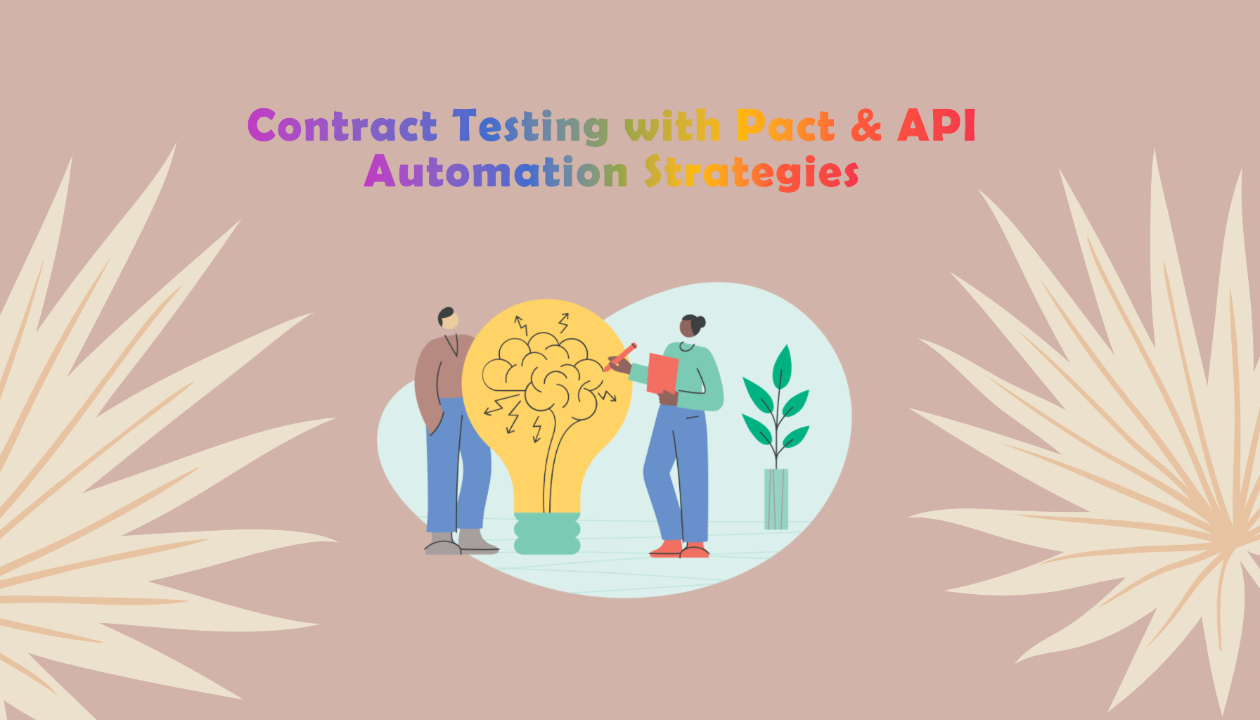
Introduction: Why Contract Testing Matters in API Development
APIs serve as the backbone of modern applications, enabling seamless communication between microservices and third-party integrations. However, as applications grow in complexity, ensuring that API interactions remain reliable becomes a challenge. Contract testing with Pact has emerged as a powerful solution to tackle this problem, enabling teams to validate API interactions efficiently.
In this guide, we’ll dive deep into contract testing with Pact, why it’s essential, how it works, and the best practices for API automation strategies to enhance software quality.
What is Contract Testing?
Contract testing is a testing methodology that ensures APIs (both consumer and provider services) communicate correctly. Instead of testing every possible API interaction through end-to-end tests, contract testing focuses on verifying that the agreements (contracts) between services are honored.
Why Contract Testing?
- ✅ Prevents API contract mismatches between microservices.
- ✅ Reduces dependency on full end-to-end testing, making CI/CD pipelines faster.
- ✅ Ensures stability and backward compatibility when API changes occur.
- ✅ Improves test reliability by eliminating flaky integration tests.
Understanding Pact: The Most Popular Contract Testing Framework
Pact is an open-source contract testing framework designed to verify interactions between API consumers and providers.
Key Concepts in Pact:
- Consumer: The application or service making API requests.
- Provider: The service responding to API requests.
- Contract (Pact file): A JSON file generated by the consumer that defines expected API interactions.
- Pact Broker: A repository for storing and sharing contract files between teams.
- Verification: The provider validates that it meets the contract expectations defined by the consumer.
How Pact Works in API Testing
- Consumer Generates a Contract: The consumer creates a test that defines expected API interactions.
- Contract is stored: The contract (Pact file) is stored in a Pact Broker.
- Provider Validates the Contract: The provider runs tests to verify compliance with the contract.
- CI/CD Integration: Automated contract testing is executed within the CI/CD pipeline to prevent breaking changes.
Setting Up Contract Testing with Pact
Step 1: Install Pact in Your Project
Depending on your tech stack, install the relevant Pact library:
- Node.js: npm install --save-dev @pact-foundation/pact
- Java: testImplementation 'au.com.dius:pact-jvm-consumer-junit:4.3.4'
- Python: pip install pact-python
Step 2: Create a Consumer Test
const { Pact } = require('@pact-foundation/pact');
const provider = new Pact({ consumer: 'FrontendApp', provider: 'UserService' });
describe('Pact Consumer Test', () => {
beforeAll(() => provider.setup());
afterAll(() => provider.finalize());
it('should return a valid user', async () => {
await provider.addInteraction({
state: 'User exists',
uponReceiving: 'A request for user data',
withRequest: { method: 'GET', path: '/user/1' },
willRespondWith: { status: 200, body: { id: 1, name: 'John Doe' } },
});
});
});
Step 3: Publish Contracts to Pact Broker
pact-broker publish pacts --broker-base-url=http://pact-broker-url --consumer-app-version=1.0.0
Step 4: Provider Verification
On the provider side, we verify that the API response matches the consumer expectations.
@RunWith(PactProviderTest.class)
@Provider("UserService")
@PactBroker(url = "http://pact-broker-url")
public class ProviderContractTest {
@TestTarget public final Target target = new HttpTarget(8080);
@State("User exists")
public void userExists() {}
}
Best Practices for API Automation with Pact
1. Use Pact Broker for Collaboration
- Store and manage contracts efficiently.
- Enable real-time contract sharing between teams.
2. Automate Contract Testing in CI/CD Pipelines
- Run Pact tests automatically in GitHub Actions, Jenkins, or GitLab CI/CD.
- Prevent the deployment of services that introduce breaking API changes.
3. Implement Consumer-Driven Testing
- Consumers should define expected API interactions.
- Providers must validate against consumer expectations.
4. Keep Contracts Small and Specific
- Define only necessary interactions in the contract.
- Avoid bloated contracts that slow down testing.
5. Combine Contract Testing with Other API Testing Strategies
- Use contract testing to verify API communication.
- Implement integration & end-to-end tests for business logic validation.
Challenges & Solutions in Contract Testing
1. Keeping Contracts Up-to-Date 🔄
Solution: Automate contract updates and enforce Pact Broker synchronization.
2. Managing Multiple Consumers & Providers 🔗
Solution: Use Pactflow or structured tagging in Pact Broker to manage dependencies.
3. Handling Dynamic API Responses ⚡
Solution: Use Pact matchers (like(), regex(), integer()) to support flexible verification.
Conclusion: Why You Should Adopt Pact for API Automation
Contract testing with Pact is a game-changer for microservices and API automation. It helps teams avoid breaking changes, speeds up CI/CD workflows, and enhances test reliability.
🔹 Reduces integration testing complexity
🔹 Enhances API reliability in microservices
🔹 Supports faster software delivery cycles
By integrating contract testing into your API automation strategy, your development team can build more resilient, scalable, and maintainable applications. 🚀
Further Reading & Learning Resources
📚 Official Documentation & Tutorials
🔗 Pact Docs: https://docs.pact.io
🔗 Pactflow (Managed Pact Broker): https://pactflow.io
🎓 Online Courses & Training
🔗 Udemy: Contract Testing with Pact https://www.udemy.com
🔗 LinkedIn Learning: API Testing Strategies https://www.linkedin.com/learning
📢 What’s Your Experience with Contract Testing?
Share your insights, and let’s discuss how Pact & API automation can improve software quality! 🚀